Description
The BitMEX Trading API is written for for NinjaTrader 8 trading platform. During installation it adds the following DLL’s to the NT8 bin/Custom folder:
- NinjaTrader.Bitmex.dll
- Newtonsoft.Json.dll
The installation also contains a demo strategy, which shows some (not all) of the API functions:
Please be aware that the provided API trading functions are not fully integrated into the NT8 environment! This means, that NT8 is not aware of a trade if executed and therewith it’s not showing up in performance statistics!
A provided solution demonstrated in the demo strategy is to call the NT8 trading functions EnterLong, EnterShort upon receiving a confirmation for the executed trade from the BitMEX trading API. This allows to simulate and visualize trades in the chart with the simulation account.
The API only executes trades if in realtime mode! If you want to backtest your strategy we would recommend to use a copy of the strategy w/o BitMEX trading API functions enabled, or disable them in the strategy e.g. with a separate “Backtest Enabled” parameter.
The API does not fully support all BitMEX API trading functions as provided by the BitMEX API website, but we are looking forward to requests to add missing functions!
After the checkout you will receive an email with a download link.
Installation
- Save the provided zip-file (no need to unzip) to the location of your choice
- Go to NinjaTrader menu Tools => Import => NinjaScript Add-On…
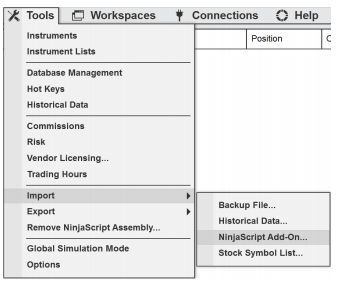
- Select the saved zip-file in the opened dialog
- NinjaTrader will guide you through the installation. At the end there should be a success message.
- Restart NinjaTrader
NOTE: If you have a previous version installed, before installing the new one, you need to uninstall the old one first!
NinjaTrader
Our Recommended Trading Platform
NinjaTrader® is our #1 recommended trading software preferred by traders worldwide including our clients.
Download NinjaTrader & receive immediate FREE access to:
■ Real-time futures data
■ Unlimited real-time forex data
■ Advanced charting
■ Trade simulator
■ Strategy development and backtesting
■ Connect to NinjaTrader Brokerage, Interactive Brokers, TD Ameritrade & more…
NinjaTrader’s award-winning trading platform is consistently voted an industry leader by the trading community. Featuring 1000s of Apps & Add-Ons for unlimited customization, NinjaTrader is used by over 60,000 traders for advanced market analysis, professional charting and fast order execution.
For new traders, start preparing for the live markets with a free Trading simulator featuring real-time market data.
Get Started For Free!
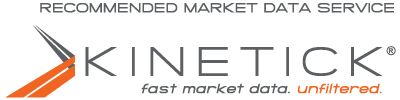
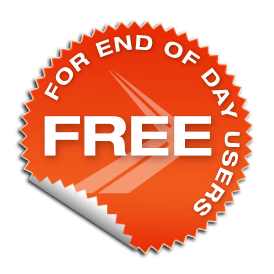
Our Recommended Market Data Feed
Kinetick® delivers reliable, fast and cost-effective market data to help level the playing field for active traders. Take advantage of unfiltered, real time quotes for stocks, futures and forex that exceed the expectations of the world’s most demanding traders, like us!
Get started with FREE end-of-day historical market data through the NinjaTrader platform and learn how you can significantly reduce CME Group Globex exchange fees on real-time market data with Kinetick.
Get Started with Free EOD Data
Version History
V1.8
Supported variables and functions:
/// <summary>
/// Set/get rate-limit defined by BitMEX.
/// </summary>
public string RateLimitMax;
/// <summary>
/// Set/get remaining rate-limit defined by BitMEX.
/// </summary>
public string RateLimitRemaining;
/// <summary>
/// API Error counter.
/// </summary>
public int ErrorCount;
/// <summary>
/// API constructor.
/// </summary>
/// <param name="printCallback">Print output function to use.</param>
/// <param name="bitmexKey">BitMEX key.</param>
/// <param name="bitmexSecret">BitMEX secret.</param>
/// <param name="testNet">Use testnet if set to true.</param>
/// <param name="rateLimit">Set default rate limit.</param>
public BitmexApi( Action<object> printCallback, string bitmexKey = "", string bitmexSecret = "", bool testNet = false, int rateLimit = 500);
/// <summary>
/// Get existing order by ID.
/// </summary>
/// <param name="order">Order reference.</param>
/// <returns>True if successfully executed, false otherwise.</returns>
public bool bool OrderGetByOrderId(ref Order order);
/// <summary>
/// Post a new stop-market order.
/// </summary>
/// <param name="order">Order reference.</param>
/// <param name="symbol">Symbol.</param>
/// <param name="side">Order side: 'Buy' or 'Sell'.</param>
/// <param name="stopPrice">Stop-price.</param>
/// <param name="quantity">Order quantity.</param>
/// <returns>True if successfully executed, false otherwise.</returns>
/// <remarks>
/// Sample request URL: https://www.bitmex.com/api/v1/order
/// Sample response:
/// {
/// "orderID": "8a0e0569-ab60-9e23-6efb-0cb7ecc003e8",
/// "clOrdID": "BuyTest",
/// "clOrdLinkID": "",
/// "account": 559459,
/// "symbol": "XBTUSD",
/// "side": "Buy",
/// "simpleOrderQty": null,
/// "orderQty": 1,
/// "price": null,
/// "displayQty": null,
/// "stopPx": 6370,
/// "pegOffsetValue": null,
/// "pegPriceType": "",
/// "currency": "USD",
/// "settlCurrency": "XBt",
/// "ordType": "Stop",
/// "timeInForce": "ImmediateOrCancel",
/// "execInst": "",
/// "contingencyType": "",
/// "exDestination": "XBME",
/// "ordStatus": "New",
/// "triggered": "",
/// "workingIndicator": false,
/// "ordRejReason": "",
/// "simpleLeavesQty": null,
/// "leavesQty": 1,
/// "simpleCumQty": null,
/// "cumQty": 0,
/// "avgPx": null,
/// "multiLegReportingType": "SingleSecurity",
/// "text": "Submitted via API.",
/// "transactTime": "2018-11-12T02:26:10.702Z",
/// "timestamp": "2018-11-12T02:26:10.702Z"
/// }
/// Response header:
/// {
/// "date": "Mon, 12 Nov 2018 02:26:10 GMT",
/// "content-encoding": "gzip",
/// "x-powered-by": "Profit",
/// "etag": "W/"2c6-Ov4r+Bh2UAE0lLZBuSSIk7Ea+L0"",
/// "x-ratelimit-remaining": "149",
/// "content-type": "application/json; charset=utf-8",
/// "status": "200",
/// "x-ratelimit-reset": "1541989571",
/// "x-ratelimit-limit": "150",
/// "strict-transport-security": "max-age=31536000; includeSubDomains"
/// }
/// </remarks>
public bool OrderPostStopMarket( ref Order order, string symbol, string side, double stopPrice, int quantity);
/// <summary>
/// Post a new stop-limit order.
/// </summary>
/// <param name="order">Order reference.</param>
/// <param name="symbol">Symbol.</param>
/// <param name="side">Order side: 'Buy' or 'Sell'.</param>
/// <param name="stopPrice">Stop-price.</param>
/// <param name="limitPrice">Limit-price.</param>
/// <param name="quantity">Order quantity.</param>
/// <returns>True if successfully executed, false otherwise.</returns>
public bool OrderPostStopLimit( ref Order order, string symbol, string side, double stopPrice, double limitPrice, int quantity);
/// <summary>
/// Post a new limit order.
/// </summary>
/// <param name="order">Order reference.</param>
/// <param name="symbol">Symbol.</param>
/// <param name="side">Order side: 'Buy' or 'Sell'.</param>
/// <param name="limitPrice">Limit-price.</param>
/// <param name="quantity">Order quantity.</param>
/// <returns>True if successfully executed, false otherwise.</returns>
public bool OrderPostLimit( ref Order order, string symbol, MarketSide side, double limitPrice, int quantity);
/// <summary>
/// Edit existing order which is not filled yet.
/// </summary>
/// <param name="order">Order reference.</param>
/// <param name="stopPrice">Stop-price.</param>
/// <param name="limitPrice">Limit-price.</param>
/// <param name="quantity">Order quantity.</param>
/// <returns>True if successfully executed, false otherwise.</returns>
public bool OrderEdit( ref Order order, double stopPrice, double limitPrice, int quantity);
/// <summary>
/// Cancel single order.
/// </summary>
/// <param name="order">Order reference.</param>
/// <returns>True if successfully executed, false otherwise.</returns>
public bool OrderCancel(ref Order order);
/// <summary>
/// Cancel all orders.
/// </summary>
/// <param name="order">Order reference.</param>
/// <returns>True if successfully executed, false otherwise.</returns>
/// <remarks>
/// Request URL: https://www.bitmex.com/api/v1/order/all
/// </remarks>
public void OrderCancelAll(string symbol);
/// <summary>
/// Get open position.
/// </summary>
/// <param name="position">Position reference.</param>
/// <param name="symbol">Symbol.</param>
/// <returns>True if successfully executed, false otherwise.</returns>
public bool PositionGet( ref Position position, string symbol);
/// <summary>
/// Get your current wallet information.
/// </summary>
/// <returns>User wallet information.</returns>
/// <remarks>
/// Request URL: https://www.bitmex.com/api/v1/user/wallet?currency=XBt
/// </remarks>
public string UserGetWallet();
Reviews
There are no reviews yet.